Understanding PHP Arrays: A Beginner's Guide to Data Structures
Written on
Chapter 1: Introduction to Arrays
In PHP, as with many programming languages, an array serves as a fundamental data structure that facilitates the organization of ordered collections of data. Arrays can contain various data types, with each element assigned a numerical index that begins at 0 and continues sequentially. This guide explores the essentials of creating and manipulating arrays in PHP as part of a comprehensive 7-part series documenting my programming journey. For additional resources, refer to the links provided at the end of this article.
Creating Arrays
PHP provides two primary methods for constructing arrays:
- Using the built-in function: array()
- Utilizing shorthand notation with square brackets.
For example, the following demonstrates these methods:
$my_array = array(1, 2, 5, 9);
$another_array = [0, 1, 2];
To determine the number of elements within an array, the count() function can be used:
echo count($my_array); // Outputs 4
Accessing Elements
To retrieve a specific element from an array, index notation is applied. Since array indexing starts at 0, to access the second element in an array named $my_array, you would use the following syntax:
$my_array = [1, 2, 3, 4];
echo $my_array[1]; // Outputs 2
Modifying Arrays
Once an array has been established, it can be modified in several ways. For example, to append an element to the end, you can use square brackets:
$my_array = [0, 1, 2];
$my_array[] = 3; // The array now becomes [0, 1, 2, 3]
To alter an existing item in the array, specify the index of the item you wish to change and assign a new value:
$my_array = [1, 2, 3, 4];
$my_array[2] = "new value"; // Now $my_array is [1, 2, "new value", 4]
Array Methods
Array methods are functions that enable us to modify or manipulate arrays more efficiently. Here are some commonly used methods:
- array_push(): This method adds one or more elements to the end of an array.
$my_array = [1, 2, 3];
array_push($my_array, 4, 5);
// Now $my_array is [1, 2, 3, 4, 5]
- array_pop(): This method removes the last element from an array.
$my_array = [1, 2, 3, 4];
array_pop($my_array);
// Now $my_array is [1, 2, 3]
- array_unshift(): This method adds one or more elements to the beginning of an array.
$my_array = [1, 2, 3];
array_unshift($my_array, 0);
// Now $my_array is [0, 1, 2, 3]
- array_shift(): This method removes the first element from an array.
$my_array = [1, 2, 3];
array_shift($my_array);
// Now $my_array is [2, 3]
Nested Arrays
As previously mentioned, arrays can store mixed data types, including other arrays. A nested array example would be:
$my_array = [[1, 2], [3, 4], [5, 6]];
To access elements in a nested array, you can use index notation for both the outer and inner arrays:
echo $my_array[1][0]; // Outputs 3
Associative Arrays
An associative array, or map, is a data structure where elements are accessed by keys rather than indices. This is akin to a dictionary where each key is linked to a corresponding value. For instance, if you wanted to maintain a list of friends' ages, an associative array would be much more intuitive:
$friends_ages = ["Bob" => 23, "Clive" => 49, "Amelia" => 15];
To access an element, use the key instead of an index:
echo $friends_ages["Bob"]; // Outputs 23
Adding a new element to an associative array involves specifying the new key and its associated value:
$friends_ages["Sara"] = 26;
// Adds a key for Sara with her age
To remove an element from an associative array, the unset() function is utilized:
unset($friends_ages["Clive"]);
// Removes Clive from the array
Finally, to update an existing value in an associative array, simply refer to the key and assign a new value:
$friends_ages["Bob"] = 24;
// Updates Bob's age to 24
For those of you embarking on your PHP learning journey, I hope this introduction proves helpful, and I invite you to follow along with the other articles in this series.
Links to Additional PHP Resources
- Should You Still Learn PHP in 2022?
- PHP 101: Fundamentals
- PHP 101: Functions
- PHP 101: Arrays
- PHP 101: Conditionals
- PHP 101: Loops (coming soon)
- PHP 101: Form Handling (coming soon)
- PHP 101: Classes and Objects (coming soon)
- PHP 101: My First Project (coming soon)
If you choose to join Medium through my link, I will receive a small commission at no extra cost to you! Thank you for your support. 💰
Thanks for reading!
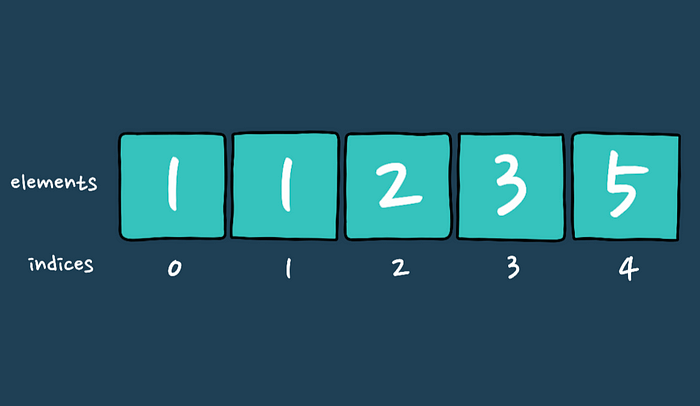
Chapter 2: PHP 101 - Arrays Part 2
In this video, we delve deeper into the intricacies of PHP arrays, exploring their various types and applications.
Chapter 3: PHP 101 - Sorting Arrays
This video focuses on the methods available for sorting arrays in PHP, showcasing practical examples and best practices.