Harnessing Neural Networks for Sentiment Analysis Insights
Written on
Understanding Sentiment Analysis
Sentiment Analysis involves the computational examination of opinions, emotions, and attitudes conveyed through written text. Essentially, it seeks to determine the emotional tone behind a given piece of writing. For instance, if someone tweets, “I love this product!” sentiment analysis would categorize this as a “positive” sentiment.
Importance of Sentiment Analysis
Why is it crucial to detect sentiments in text? Here are a few reasons:
- Business Insights: Organizations leverage sentiment analysis to gauge customer feedback and enhance their offerings.
- Market Research: It aids in capturing public sentiment regarding a specific issue, such as an election or product launch.
- Social Media Monitoring: Analyze public reactions to trending topics or news. Are people feeling angry, happy, or indifferent?
- Customer Service: Automatically categorizing customer inquiries based on sentiment helps prioritize urgent requests.
- Personal Projects: This analysis can be utilized in various applications, from mood-tracking diaries to dating apps assessing icebreaker success.
How Sentiment Analysis Works
Previously, sentiment analysis relied on straightforward keyword matching or rule-based systems. However, these methods have limitations due to the complexity of language. A single word can express different sentiments based on context. For example, “sick” can mean “ill” or “awesome” depending on usage.
This is where Machine Learning, particularly Neural Networks, becomes valuable. These sophisticated techniques can grasp context, sarcasm, and even colloquial expressions, enhancing the effectiveness of sentiment analysis.
Types of Sentiment Analysis
- Fine-grained Sentiment Analysis: This approach categorizes sentiments into more detailed classes, such as “very positive” or “somewhat negative.”
- Aspect-based Sentiment Analysis: Instead of evaluating the sentiment of an entire sentence, it focuses on sentiment related to specific features. For example, in the sentence “The phone has a great camera but a poor battery,” the sentiment is positive for the camera and negative for the battery.
- Emotion Analysis: This deeper analysis identifies specific emotions like joy, frustration, or anger.
Why Choose Neural Networks?
Traditional sentiment analysis methods often utilize word counts or basic algorithms to identify positive or negative words. Although somewhat effective, these approaches struggle to capture nuanced meanings and contextual relevance. In contrast, neural networks excel at learning from data, recognizing patterns, and interpreting the complexities of human language. If you're venturing into sentiment analysis, neural networks are the optimal choice!
Preparing the Data
For our example, we’ll utilize the IMDB dataset, which contains movie reviews labeled as either positive or negative. This dataset can be conveniently accessed through TensorFlow’s Keras API.
Here's how to load it:
from tensorflow.keras.datasets import imdb
# Load dataset
(train_data, train_labels), (test_data, test_labels) = imdb.load_data(num_words=10000)
The IMDB dataset consists of 50,000 movie reviews sourced from the Internet Movie Database (IMDB), categorized as either “positive” or “negative” based on the reviewer's sentiment. It is commonly employed for training and testing in sentiment analysis.
The dataset is divided into two equal portions:
- Training Data: 25,000 reviews for training the machine learning model.
- Testing Data: Another 25,000 reviews for assessing the model’s performance.
The IMDB dataset comes preprocessed to some extent:
- Tokenization: Each review is tokenized into sequences of integers.
- Limited Vocabulary: By specifying num_words=10000 when loading the dataset, we focus on the 10,000 most frequently used words, excluding rare words that may not contribute significantly.
Data Preprocessing
Before inputting the data into a neural network, preprocessing is essential. We’ll convert the integer sequences into binary matrices.
import numpy as np
def vectorize_sequences(sequences, dimension=10000):
results = np.zeros((len(sequences), dimension))
for i, sequence in enumerate(sequences):
results[i, sequence] = 1.return results
# Vectorize the data
x_train = vectorize_sequences(train_data)
x_test = vectorize_sequences(test_data)
y_train = np.asarray(train_labels).astype('float32')
y_test = np.asarray(test_labels).astype('float32')
Building the Neural Network Model
Let’s create a basic neural network with three layers using TensorFlow and Keras.
from tensorflow.keras import models, layers
model = models.Sequential([
layers.Dense(16, activation='relu', input_shape=(10000,)),
layers.Dense(16, activation='relu'),
layers.Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
Training the Model
Now, it’s time for the exciting part! Let’s train our model on the prepared data.
# Train the model
history = model.fit(x_train,
y_train,
epochs=20,
batch_size=512,
validation_split=0.2)
Evaluating and Testing
After training, we can evaluate the model's performance on the test data.
# Evaluate the model
results = model.evaluate(x_test, y_test)
print(results)
The output will display something like [0.2940, 0.8832], where the first value indicates loss and the second denotes accuracy on the test set.
- Loss (0.2940): A relatively low loss value indicates that the model’s predictions are close to the actual sentiments.
- Accuracy (0.8832 or 88.32%): This signifies that the model correctly identified the sentiment of the review approximately 88% of the time on the test set.
Real-world Application
Suppose you want to determine the sentiment of the following phrase: “This movie is absolutely fantastic!” You would preprocess this sentence similarly to our dataset and then utilize the predict function.
# Assume text_to_sequence converts the input text into the necessary format
input_data = vectorize_sequences([text_to_sequence("This movie is absolutely fantastic!")])
# Predict
prediction = model.predict(input_data)
print("Positive" if prediction >= 0.5 else "Negative")
In this scenario, it would output: Positive.
Conclusion
Neural networks provide a versatile and effective means for conducting sentiment analysis. While the model demonstrated here is relatively straightforward, you can easily expand it with additional layers, different architectures like LSTMs or Transformers, and extra features to enhance accuracy. Feel free to modify, experiment, and enjoy the process!
A Message from AI Mind
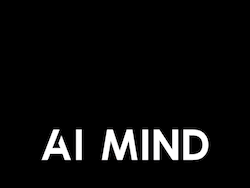
Thank you for being part of our community! Before you leave, consider:
- 👍 Applauding this article and following the author.
- 📖 Exploring more content in the AI Mind Publication.
- ✨ Effortlessly enhancing your AI prompts for free.
- 🛠️ Discovering intuitive AI tools.
Chapter 2: Practical Insights into Sentiment Analysis
In this video, we explore sentiment analysis using BERT Neural Network with Python, detailing its applications and benefits.
Chapter 3: Advanced Techniques in Sentiment Analysis
This video delves into a deep learning project on sentiment analysis using LSTM on IMDB reviews, showcasing advanced methodologies.